I need help pinpointing a problem within or after the USB enumeration process with Win10 (using usbaudio2.sys driver).
I am developing a software for the XU208-128-QF48-C10 which should get an 8-channel audio stream via usb and via tdm, mix them, and send the result via usb back to the host and via tdm to a DAC. Additionally it should receive and send MIDI data via usb from and to the host. USB should use audio class 2 and HS for the connection.
I use the latest xud library (v2.1.0) and the sw_usb_audio_6.15.2 sample as a template.
When testing, the host issues an 'URB_FUNCTION_ABORT_PIPE' (recorded with wireshark, see below) within or after the enumeration process with no communication afterwards. I can't pinpoint why the host behaves like this, thus this post :)
Following a screenshot from Wireshark. I guess the bulk data transfer at the start comes from the flashing process:
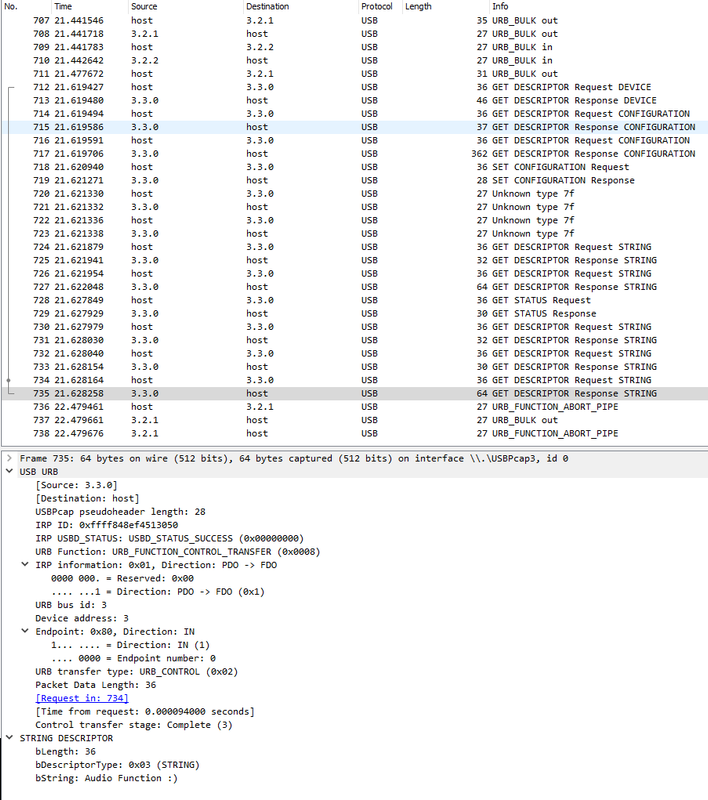
Following my usb device and config descriptors:
Code: Select all
#ifndef _USB_DESCRIPTORS_H_
#define _USB_DESCRIPTORS_H_
#include "config.h"
#include <stdint.h>
#include "descriptor_audio_def.h"
#include "descriptor_std_def.h"
#include "descriptor_values.h"
#include "usb_enum.h"
#ifndef USB_BCD_DEVICE
#define USB_BCD_DEVICE 0x0000
#warning BCD_DEVICE not defined, falling back to 0x0000
#endif
#ifndef USB_LANG_ID
#define USB_LANG_ID "\x09\x04"
#warning LANG_ID nod defined, falling back to US English
#endif
#ifndef USB_PRODUCT_ID
#define USB_PRODUCT_ID 0x0011
#warning PRODUCT_ID not defined, falling back to 0x0001
#endif
#ifndef USB_VENDOR_ID
#define USB_VENDOR_ID 0x20B1
#warning VENDOR_ID not defined, falling back to XMOS vendor ID
#endif
#define MIDI_LENGTH 92
#define RESOLUTION_BITS 32
#define MAX_FREQ 48000
#define NUM_USB_CHANS 8
#define USB_SUBSLOT_SIZE 4
// (MAX_FREQ + 7999) to guarantee rounding up
// / 8000 to calculate Packet Size per USB Microframe (125us)
// +1 to accomodate for accumulation of 'rounding error'
// (NUM_USB_CHANS * USB_SUBSLOT_SIZE) get the size of one Frame in Bytes
#define USB_MAX_PACKET_SIZE_HS ((((MAX_FREQ + 7999)/8000)+1) * (NUM_USB_CHANS * USB_SUBSLOT_SIZE))
enum USB_STRING_DESC_INDEX {
USB_CONFIG_STR_INDEX = 1,
USB_MANUFACTURER_STR_INDEX,
USB_PRODUCT_STR_INDEX,
USB_SERIAL_NO_STR_INDEX,
USB_AUDIO_FUNCTION_STR_INDEX,
USB_AUDIO_CTRL_IF_STR_INDEX,
USB_AUDIO_CLOCK_SRC_STR_INDEX,
USB_AUDIO_USB_FROM_HOST_STR_INDEX,
USB_AUDIO_TDM_FROM_DAC_STR_INDEX,
USB_AUDIO_USB_TO_HOST_STR_INDEX,
USB_AUDIO_TDM_TO_DAC_STR_INDEX,
USB_AUDIO_MIXER_STR_INDEX,
USB_AUDIO_FROM_HOST_STREAM_ALT0_STR_INDEX,
USB_AUDIO_FROM_HOST_STREAM_ALT1_STR_INDEX,
USB_AUDIO_TO_HOST_STREAM_ALT0_STR_INDEX,
USB_AUDIO_TO_HOST_STREAM_ALT1_STR_INDEX,
USB_MIDI_FROM_HOST_JACK_STR_INDEX,
USB_MIDI_FROM_ESP_JACK_STR_INDEX,
USB_MIDI_TO_HOST_JACK_STR_INDEX,
USB_MIDI_TO_ESP_JACK_STR_INDEX,
};
// USB String descriptor for the Device
static char *usb_stringDescriptors[] = {
USB_LANG_ID, // Language ID string (US English)
USB_CONFIG_DESC, // iConfiguration
USB_MANUFACTURER_DESC, // iManufacturer
USB_PRODUCT_DESC, // iProduct
USB_SERIAL_NO_DESC, // iSerial
USB_AUDIO_FUNCTION_DESC, // iFunction
USB_AUDIO_CTRL_IF_DESC,
USB_AUDIO_CLOCK_SRC_DESC,
USB_AUDIO_USB_FROM_HOST_DESC,
USB_AUDIO_TDM_FROM_DAC_DESC,
USB_AUDIO_USB_TO_HOST_DESC,
USB_AUDIO_TDM_TO_DAC_DESC,
USB_AUDIO_MIXER_DESC,
USB_AUDIO_FROM_HOST_STREAM_ALT0_DESC,
USB_AUDIO_FROM_HOST_STREAM_ALT1_DESC,
USB_AUDIO_TO_HOST_STREAM_ALT0_DESC,
USB_AUDIO_TO_HOST_STREAM_ALT1_DESC,
USB_MIDI_FROM_HOST_JACK_DESC,
USB_MIDI_FROM_ESP_JACK_DESC,
USB_MIDI_TO_HOST_JACK_DESC,
USB_MIDI_TO_ESP_JACK_DESC,
};
USB_Descriptor_Dev_t devDesc_Audio = { // OK with Sample
.bLength = sizeof(USB_Descriptor_Dev_t),
.bDescriptorType = USB_DESCTYPE_DEVICE,
.bcdUSB = USB_BCD_2,
.bDeviceClass = 0xEF,
.bDeviceSubClass = 0x02,
.bDeviceProtocol = 0x01,
.bMaxPacketSize0 = 64,
.idVendor = USB_VENDOR_ID,
.idProduct = USB_PRODUCT_ID,
.bcdDevice = USB_BCD_DEVICE,
.iManufacturer = USB_MANUFACTURER_STR_INDEX,
.iProduct = USB_PRODUCT_STR_INDEX,
.iSerialNumber = USB_SERIAL_NO_STR_INDEX,
.bNumConfigurations = 0x02, // SAMPLE: Set to 2 such that windows does not load composite driver
};
typedef struct {
USB_Descriptor_Cfg_t cfg_header;
USB_Descriptor_IF_Association_t interface_association;
// Audio Control Interface
USB_Descriptor_IF_t audio_control_std_interface;
USB_Audio_Descriptor_IF_AC_t audio_control_class_interface;
USB_Audio_Descriptor_Clock_Source_t bclk_source_unit;
USB_Audio_Descriptor_Input_Terminal_t usb_from_host_input_terminal;
USB_Audio_Descriptor_Input_Terminal_t tdm_from_adc_input_terminal_desc;
USB_Audio_Descriptor_Output_Terminal_t usb_to_host_output_terminal;
USB_Audio_Descriptor_Output_Terminal_t tdm_to_dac_output_terminal;
USB_Audio_Descriptor_Mixer_t mixer_unit;
// Audio Stream Interface 1 (Host to Device)
USB_Descriptor_IF_t audio_stream_from_host_alt0_std_interface;
USB_Descriptor_IF_t audio_stream_from_host_alt1_std_interface;
USB_Audio_Descriptor_IF_AS_t audio_stream_from_host_class_interface;
USB_Audio_Descriptor_Format_1_t audio_stream_form_host_format;
USB_Descriptor_EP_t audio_stream_from_host_std_endpoint;
USB_Audio_Descriptor_EP_AS_t audio_stream_from_host_class_endpoint;
// Audio Stream Interface 2 (Device to Host)
USB_Descriptor_IF_t audio_stream_to_host_alt0_std_interface;
USB_Descriptor_IF_t audio_stream_to_host_alt1_std_interface;
USB_Audio_Descriptor_IF_AS_t audio_stream_to_host_class_interface;
USB_Audio_Descriptor_Format_1_t audio_stream_to_host_format;
USB_Descriptor_EP_t audio_stream_to_host_std_endpoint;
USB_Audio_Descriptor_EP_AS_t audio_stream_to_host_class_endpoint;
// MIDI
uint8_t configDesc_Midi[MIDI_LENGTH];
} cfg_descriptor;
#define USB_AUDIO_CLASS_INTERFACE_LENGTH ( \
sizeof(USB_Audio_Descriptor_IF_AC_t) \
+ sizeof(USB_Audio_Descriptor_Clock_Source_t) \
+ sizeof(USB_Audio_Descriptor_Input_Terminal_t) \
+ sizeof(USB_Audio_Descriptor_Input_Terminal_t) \
+ sizeof(USB_Audio_Descriptor_Output_Terminal_t) \
+ sizeof(USB_Audio_Descriptor_Output_Terminal_t) \
+ sizeof(USB_Audio_Descriptor_Mixer_t) \
)
#define USB_MIDI_CLASS_AC_INTERFACE_LENGTH ( \
sizeof(USB_MIDI_Descriptor_IF_AC_t) \
)
#define USB_CFG_LENGTH ( \
sizeof(USB_Descriptor_Cfg_t) \
+ sizeof(USB_Descriptor_IF_Association_t) \
+ sizeof(USB_Descriptor_IF_t) \
+ USB_AUDIO_CLASS_INTERFACE_LENGTH \
/* USB Audio IN (From Host) */ \
+ sizeof(USB_Descriptor_IF_t) \
+ sizeof(USB_Descriptor_IF_t) \
+ sizeof(USB_Audio_Descriptor_IF_AS_t) \
+ sizeof(USB_Audio_Descriptor_Format_1_t) \
+ sizeof(USB_Descriptor_EP_t) \
+ sizeof(USB_Audio_Descriptor_EP_AS_t) \
/* USB Audio Out (To Host) */ \
+ sizeof(USB_Descriptor_IF_t) \
+ sizeof(USB_Descriptor_IF_t) \
+ sizeof(USB_Audio_Descriptor_IF_AS_t) \
+ sizeof(USB_Audio_Descriptor_Format_1_t) \
+ sizeof(USB_Descriptor_EP_t) \
+ sizeof(USB_Audio_Descriptor_EP_AS_t) \
/* USB MIDI */ \
+ MIDI_LENGTH \
)
cfg_descriptor cfgDesc_Audio = { // OK with Sample
.cfg_header = {
.bLength = (uint8_t)sizeof(USB_Descriptor_Cfg_t),
.bDescriptorType = USB_DESCTYPE_CONFIGURATION,
.wTotalLength = USB_CFG_LENGTH,
.bNumInterfaces = USB_INTERFACE_COUNT,
.bConfigurationValue = 1,
.iConfiguration = USB_CONFIG_STR_INDEX,
#ifdef SELF_POWERED
.bmAttributes = 0xC0,
#else
.bmAttributes = 0x80,
#endif
.bMaxPower = BMAX_POWER,
},
.interface_association = { // OK with Sample
.bLength = (uint8_t)sizeof(USB_Descriptor_IF_Association_t),
.bDescriptorType = USB_DESCTYPE_INTERFACE_ASSOCIATION,
.bFirstInterface = 0x00,
.bInterfaceCount = USB_INTERFACE_COUNT,
.bFunctionClass = USB_INTERFACE_CLASS_AUDIO,
.bFunctionSubClass = USB_INTERFACE_SUBCLASS_UNDEFINED,
.bFunctionProtocol = USB_INTERFACE_PROTOCOL_2,
.iFunction = USB_AUDIO_FUNCTION_STR_INDEX,
},
.audio_control_std_interface = { // OK with Sample
.bLength = (uint8_t)sizeof(USB_Descriptor_IF_t),
.bDescriptorType = USB_DESCTYPE_INTERFACE,
.bInterfaceNumber = USB_INTERFACE_NUMBER_AUDIO_CONTROL,
.bAlternateSetting = 0x00, // must be 0 for AC
.bNumEndpoints = 0x00, // EP 0 is not counted here
.bInterfaceClass = USB_INTERFACE_CLASS_AUDIO,
.bInterfaceSubClass = USB_INTERFACE_SUBCLASS_CONTROL,
.bInterfaceProtocol = USB_INTERFACE_PROTOCOL_2,
.iInterface = USB_AUDIO_CTRL_IF_STR_INDEX,
},
.audio_control_class_interface = { // OK with Sample
.bLength = (uint8_t)sizeof(USB_Audio_Descriptor_IF_AC_t),
.bDescriptorType = USB_AUDIO_DESCTYPE_INTERFACE,
.bDescriptorSubtype = USB_AUDIO_IF_AC_SUBTYPE_HEADER,
.bcdADC = 0x0200, // compliant with USB2
.bCategory = 0x0A, // Pro Audio
.wTotalLength = USB_AUDIO_CLASS_INTERFACE_LENGTH,
.bmControls = 0x00,
},
.bclk_source_unit = { // OK with Sample
.bLength = (uint8_t)sizeof(USB_Audio_Descriptor_Clock_Source_t),
.bDescriptorType = USB_AUDIO_DESCTYPE_INTERFACE,
.bDescriptorSubtype = USB_AUDIO_IF_AC_SUBTYPE_CLOCK_SOURCE,
.bClockID = USB_ID_BCLK,
.bmAttributes = 0x00,
.bmControls = 0x00, // SAMPLE: 0x07
.bAssocTerminal = USB_ID_NONE,
.iClockSource = USB_AUDIO_CLOCK_SRC_STR_INDEX,
},
.usb_from_host_input_terminal = { // OK with Sample
.bLength = (uint8_t)sizeof(USB_Audio_Descriptor_Input_Terminal_t),
.bDescriptorType = USB_AUDIO_DESCTYPE_INTERFACE,
.bDescriptorSubtype = USB_AUDIO_IF_AC_SUBTYPE_INPUT_TERMINAL,
.bTerminalID = USB_ID_USB_FROM_HOST,
.wTerminalType = 0x0101, // USB Streaming
.bAssocTerminal = 0x00,
.bCSourceID = USB_ID_BCLK,
.audio_cluster =
{
.bNrChannels = NUM_USB_CHANS,
.bmChannelConfig = 0x000000FF,
.iChannelNames = 0,
},
.bmControls = 0x0000,
.iTerminal = USB_AUDIO_USB_FROM_HOST_STR_INDEX,
},
.tdm_from_adc_input_terminal_desc = { // OK with Sample
.bLength = (uint8_t)sizeof(USB_Audio_Descriptor_Input_Terminal_t),
.bDescriptorType = USB_AUDIO_DESCTYPE_INTERFACE,
.bDescriptorSubtype = USB_AUDIO_IF_AC_SUBTYPE_INPUT_TERMINAL,
.bTerminalID = USB_ID_TDM_FROM_ADC,
.wTerminalType = 0x0200, // Input undefined
.bAssocTerminal = 0x00,
.bCSourceID = USB_ID_BCLK,
.audio_cluster =
{
.bNrChannels = NUM_USB_CHANS,
.bmChannelConfig = 0x000000FF,
.iChannelNames = 0,
},
.bmControls = 0x0000,
.iTerminal = USB_AUDIO_TDM_FROM_DAC_STR_INDEX,
},
.usb_to_host_output_terminal = { // OK with Sample
.bLength = (uint8_t)sizeof(USB_Audio_Descriptor_Output_Terminal_t),
.bDescriptorType = USB_AUDIO_DESCTYPE_INTERFACE,
.bDescriptorSubtype = USB_AUDIO_IF_AC_SUBTYPE_OUTPUT_TERMINAL,
.bTerminalID = USB_ID_USB_TO_HOST,
.wTerminalType = 0x0101, // USB Streaming
.bAssocTerminal = 0,
.bSourceID = USB_ID_MIXER,
.bCSourceID = USB_ID_BCLK,
.bmControls = 0,
.iTerminal = USB_AUDIO_USB_TO_HOST_STR_INDEX,
},
.tdm_to_dac_output_terminal = { // OK with Sample
.bLength = (uint8_t)sizeof(USB_Audio_Descriptor_Output_Terminal_t),
.bDescriptorType = USB_AUDIO_DESCTYPE_INTERFACE,
.bDescriptorSubtype = USB_AUDIO_IF_AC_SUBTYPE_OUTPUT_TERMINAL,
.bTerminalID = USB_ID_TDM_FROM_ADC,
.wTerminalType = 0x0301, // Output to Speaker
.bAssocTerminal = 0,
.bSourceID = USB_ID_MIXER,
.bCSourceID = USB_ID_BCLK,
.bmControls = 0,
.iTerminal = USB_AUDIO_TDM_TO_DAC_STR_INDEX,
},
.mixer_unit = {
.bLength = sizeof(USB_Audio_Descriptor_Mixer_t),
.bDescriptorType = USB_AUDIO_DESCTYPE_INTERFACE,
.bDescriptorSubtype = USB_AUDIO_IF_AC_SUBTYPE_MIXER_UNIT,
.bUnitID = USB_ID_MIXER, // Unique Unit-ID of the Terminal
.bNrInPins = 2, // Number of Input Pins of this unit
.baSourceID1 = USB_ID_USB_FROM_HOST,
.baSourceID2 = USB_ID_TDM_FROM_ADC,
.audio_cluster = {
.bNrChannels = NUM_USB_CHANS,
.bmChannelConfig = 0x000000FF,
.iChannelNames = 0,
},
.bmMixerControlsInput1 = 0x0000000000000000,
.bmMixerControlsInput2 = 0x0000000000000000,
.bmControls = 0,
.iMixer = USB_AUDIO_MIXER_STR_INDEX,
},
/* USB Stream from Host */
.audio_stream_from_host_alt0_std_interface = { // OK with Sample
.bLength = (uint8_t)sizeof(USB_Descriptor_IF_t),
.bDescriptorType = USB_DESCTYPE_INTERFACE,
.bInterfaceNumber = USB_INTERFACE_NUMBER_AUDIO_FROM_HOST,
.bAlternateSetting = 0,
.bNumEndpoints = 0,
.bInterfaceClass = USB_INTERFACE_CLASS_AUDIO,
.bInterfaceSubClass = USB_INTERFACE_SUBCLASS_STREAMING,
.bInterfaceProtocol = USB_INTERFACE_PROTOCOL_2,
.iInterface = USB_AUDIO_FROM_HOST_STREAM_ALT0_STR_INDEX,
},
.audio_stream_from_host_alt1_std_interface = { // OK with Sample
.bLength = (uint8_t)sizeof(USB_Descriptor_IF_t),
.bDescriptorType = USB_DESCTYPE_INTERFACE,
.bInterfaceNumber = USB_INTERFACE_NUMBER_AUDIO_FROM_HOST,
.bAlternateSetting = 1,
.bNumEndpoints = 1,
.bInterfaceClass = USB_INTERFACE_CLASS_AUDIO,
.bInterfaceSubClass = USB_INTERFACE_SUBCLASS_STREAMING,
.bInterfaceProtocol = USB_INTERFACE_PROTOCOL_2,
.iInterface = USB_AUDIO_FROM_HOST_STREAM_ALT1_STR_INDEX,
},
.audio_stream_from_host_class_interface = { // OK with Sample
.bLength = (uint8_t)sizeof(USB_Audio_Descriptor_IF_AS_t),
.bDescriptorType = USB_AUDIO_DESCTYPE_INTERFACE,
.bDescriptorSubType = USB_AUDIO_IF_AS_SUBTYPE_GENERAL,
.bTerminalLink = USB_ID_USB_FROM_HOST,
.bmControls = 0x00,
.bFormatType = 0x01,
.bmFormats = 0x00000001,
.audio_cluster =
{
.bNrChannels = NUM_USB_CHANS,
.bmChannelConfig = 0x000000FF,
.iChannelNames = 0x00,
},
},
.audio_stream_form_host_format = { // OK with Sample
.bLength = (uint8_t)sizeof(USB_Audio_Descriptor_Format_1_t),
.bDescriptorType = USB_AUDIO_DESCTYPE_INTERFACE,
.bDescriptorSubtype = USB_AUDIO_IF_AS_SUBTYPE_FORMAT_TYPE,
.bFormatType = 1,
.bSubSlotSize = USB_SUBSLOT_SIZE,
.bBitResolution = RESOLUTION_BITS,
},
.audio_stream_from_host_std_endpoint = { // OK with Sample
.bLength = (uint8_t) sizeof(USB_Descriptor_EP_t),
.bDescriptorType = USB_DESCTYPE_ENDPOINT,
.bEndpointAddress = 1,
.bmAttributes = 0x05,
.wMaxPacketSize = (uint16_t)USB_MAX_PACKET_SIZE_HS,
.bInterval = 1,
},
.audio_stream_from_host_class_endpoint = { // OK with Sample
.bLength = (uint8_t) sizeof(USB_Audio_Descriptor_EP_AS_t),
.bDescriptorType = USB_AUDIO_DESCTYPE_ENDPOINT,
.bDescriptorSubtype = USB_AUDIO_IF_AS_SUBTYPE_GENERAL,
.bmAttributes = 0x00,
.bmControls = 0x00,
.bLockDelayUnits = 0x02,
.wLockDelay = 0x0008,
},
/* USB Stream To Host */
.audio_stream_to_host_alt0_std_interface = { // OK with Sample
.bLength = (uint8_t)sizeof(USB_Descriptor_IF_t),
.bDescriptorType = USB_DESCTYPE_INTERFACE,
.bInterfaceNumber = USB_INTERFACE_NUMBER_AUDIO_TO_HOST,
.bAlternateSetting = 0,
.bNumEndpoints = 0,
.bInterfaceClass = USB_INTERFACE_CLASS_AUDIO,
.bInterfaceSubClass = USB_INTERFACE_SUBCLASS_STREAMING,
.bInterfaceProtocol = USB_INTERFACE_PROTOCOL_2,
.iInterface = USB_AUDIO_TO_HOST_STREAM_ALT0_STR_INDEX,
},
.audio_stream_to_host_alt1_std_interface = { // OK with Sample
.bLength = (uint8_t)sizeof(USB_Descriptor_IF_t),
.bDescriptorType = USB_DESCTYPE_INTERFACE,
.bInterfaceNumber = USB_INTERFACE_NUMBER_AUDIO_TO_HOST,
.bAlternateSetting = 1,
.bNumEndpoints = 1,
.bInterfaceClass = USB_INTERFACE_CLASS_AUDIO,
.bInterfaceSubClass = USB_INTERFACE_SUBCLASS_STREAMING,
.bInterfaceProtocol = USB_INTERFACE_PROTOCOL_2,
.iInterface = USB_AUDIO_TO_HOST_STREAM_ALT1_STR_INDEX,
},
.audio_stream_to_host_class_interface = { // OK with Sample
.bLength = (uint8_t)sizeof(USB_Audio_Descriptor_IF_AS_t),
.bDescriptorType = USB_AUDIO_DESCTYPE_INTERFACE,
.bDescriptorSubType = USB_AUDIO_IF_AS_SUBTYPE_GENERAL,
.bTerminalLink = USB_ID_USB_TO_HOST,
.bmControls = 0,
.bFormatType = 1,
.bmFormats = 0x00000001,
.audio_cluster =
{
.bNrChannels = NUM_USB_CHANS,
.bmChannelConfig = 0x000000FF,
.iChannelNames = 0x00,
},
},
.audio_stream_to_host_format = { // OK with Sample
.bLength = (uint8_t)sizeof(USB_Audio_Descriptor_Format_1_t),
.bDescriptorType = USB_AUDIO_DESCTYPE_INTERFACE,
.bDescriptorSubtype = USB_AUDIO_IF_AS_SUBTYPE_FORMAT_TYPE,
.bFormatType = 1,
.bSubSlotSize = USB_SUBSLOT_SIZE,
.bBitResolution = RESOLUTION_BITS,
},
.audio_stream_to_host_std_endpoint = { // OK with Sample
.bLength = (uint8_t)sizeof(USB_Descriptor_EP_t),
.bDescriptorType = USB_DESCTYPE_ENDPOINT,
.bEndpointAddress = 1,
.bmAttributes = 0x05, // SAMPLE: 0x25: Iso, async, implicit feedback data endpoint
.wMaxPacketSize = USB_MAX_PACKET_SIZE_HS,
.bInterval = 0x01,
},
.audio_stream_to_host_class_endpoint = { // OK with Sample
.bLength = (uint8_t)sizeof(USB_Audio_Descriptor_EP_AS_t),
.bDescriptorType = USB_AUDIO_DESCTYPE_ENDPOINT,
.bDescriptorSubtype = USB_AUDIO_IF_AS_SUBTYPE_GENERAL,
.bmAttributes = 0,
.bmControls = 0,
.bLockDelayUnits = 02,
.wLockDelay = 0x0008,
},
// MIDI (taken from sample)
/* MIDI Descriptors */
/* Table B-3: MIDI Adapter Standard AC Interface Descriptor */
{0x09, /* 0 bLength : Size of this descriptor, in bytes. (field size 1 bytes) */
0x04, /* 1 bDescriptorType : INTERFACE descriptor. (field size 1 bytes) */
USB_INTERFACE_NUMBER_MIDI_CONTROL, /* 2 bInterfaceNumber : Index of this interface. (field size 1 bytes) */
0x00, /* 3 bAlternateSetting : Index of this setting. (field size 1 bytes) */
0x00, /* 4 bNumEndpoints : 0 endpoints. (field size 1 bytes) */
0x01, /* 5 bInterfaceClass : AUDIO. (field size 1 bytes) */
0x01, /* 6 bInterfaceSubclass : AUDIO_CONTROL. (field size 1 bytes) */
0x00, /* 7 bInterfaceProtocol : Unused. (field size 1 bytes) */
0x00, /* 8 iInterface : Unused. (field size 1 bytes) */
/* Table B-4: MIDI Adapter Class-specific AC Interface Descriptor */
0x09, /* 0 bLength : Size of this descriptor, in bytes. (field size 1 bytes) */
0x24, /* 1 bDescriptorType : 0x24. (field size 1 bytes) */
0x01, /* 2 bDescriptorSubtype : HEADER subtype. (field size 1 bytes) */
0x00, /* 3 bcdADC : Revision of class specification - 1.0 (field size 2 bytes) */
0x01, /* 4 bcdADC */
0x09, /* 5 wTotalLength : Total size of class specific descriptors. (field size 2 bytes) */
0x00, /* 6 wTotalLength */
0x01, /* 7 bInCollection : Number of streaming interfaces. (field size 1 bytes) */
0x01, /* 8 baInterfaceNr(1) : MIDIStreaming interface 1 belongs to this AudioControl interface */
/* Table B-5: MIDI Adapter Standard MS Interface Descriptor */
0x09, /* 0 bLength : Size of this descriptor, in bytes. (field size 1 bytes) */
0x04, /* 1 bDescriptorType : INTERFACE descriptor. (field size 1 bytes) */
USB_INTERFACE_NUMBER_MIDI_STREAM, /* 2 bInterfaceNumber : Index of this interface. (field size 1 bytes) */
0x00, /* 3 bAlternateSetting : Index of this alternate setting. (field size 1 bytes) */
0x02, /* 4 bNumEndpoints : 2 endpoints. (field size 1 bytes) */
0x01, /* 5 bInterfaceClass : AUDIO. (field size 1 bytes) */
0x03, /* 6 bInterfaceSubclass : MIDISTREAMING. (field size 1 bytes) */
0x00, /* 7 bInterfaceProtocol : Unused. (field size 1 bytes) */
0x00, /* 8 iInterface : Unused. (field size 1 bytes) */
/* Table B-6: MIDI Adapter Class-specific MS Interface Descriptor */
0x07, /* 0 bLength : Size of this descriptor, in bytes. (field size 1 bytes) */
0x24, /* 1 bDescriptorType : CS_INTERFACE. (field size 1 bytes) */
0x01, /* 2 bDescriptorSubtype : MS_HEADER subtype. (field size 1 bytes) */
0x00, /* 3 BcdADC : Revision of this class specification. (field size 2 bytes) */
0x01, /* 4 BcdADC */
0x41, /* 5 wTotalLength : Total size of class-specific descriptors. (field size 2 bytes) */
0x00, /* 6 wTotalLength */
/* Table B-7: MIDI Adapter MIDI IN Jack Descriptor (Embedded) */
0x06, /* 0 bLength : Size of this descriptor, in bytes. (field size 1 bytes) */
0x24, /* 1 bDescriptorType : CS_INTERFACE. (field size 1 bytes) */
0x02, /* 2 bDescriptorSubtype : MIDI_IN_JACK subtype. (field size 1 bytes) */
0x01, /* 3 bJackType : EMBEDDED. (field size 1 bytes) */
0x01, /* 4 bJackID : ID of this Jack. (field size 1 bytes) */
0x00, /* 5 iJack : Unused. (field size 1 bytes) */
/* Table B-8: MIDI Adapter MIDI IN Jack Descriptor (External) */
0x06, /* 0 bLength : Size of this descriptor, in bytes. (field size 1 bytes) */
0x24, /* 1 bDescriptorType : CS_INTERFACE. (field size 1 bytes) */
0x02, /* 2 bDescriptorSubtype : MIDI_IN_JACK subtype. (field size 1 bytes) */
0x02, /* 3 bJackType : EXTERNAL. (field size 1 bytes) */
0x02, /* 4 bJackID : ID of this Jack. (field size 1 bytes) */
USB_MIDI_FROM_ESP_JACK_STR_INDEX, /* 5 iJack : Unused. (field size 1 bytes) */
/* Table B-9: MIDI Adapter MIDI OUT Jack Descriptor (Embedded) */
0x09, /* 0 bLength : Size of this descriptor, in bytes. (field size 1 bytes) */
0x24, /* 1 bDescriptorType : CS_INTERFACE. (field size 1 bytes) */
0x03, /* 2 bDescriptorSubtype : MIDI_OUT_JACK subtype. (field size 1 bytes) */
0x01, /* 3 bJackType : EMBEDDED. (field size 1 bytes) */
0x03, /* 4 bJackID : ID of this Jack. (field size 1 bytes) */
0x01, /* 5 bNrInputPins : Number of Input Pins of this Jack. (field size 1 bytes) */
0x02, /* 6 BaSourceID(1) : ID of the Entity to which this Pin is connected. (field size 1 bytes) */
0x01, /* 7 BaSourcePin(1) : Output Pin number of the Entityt o which this Input Pin is connected. */
0x00, /* 8 iJack : Unused. (field size 1 bytes) */
/* Table B-10: MIDI Adapter MIDI OUT Jack Descriptor (External) */
0x09, /* 0 bLength : Size of this descriptor, in bytes. (field size 1 bytes) */
0x24, /* 1 bDescriptorType : CS_INTERFACE. (field size 1 bytes) */
0x03, /* 2 bDescriptorSubtype : MIDI_OUT_JACK subtype. (field size 1 bytes) */
0x02, /* 3 bJackType : EXTERNAL. (field size 1 bytes) */
0x04, /* 4 bJackID : ID of this Jack. (field size 1 bytes) */
0x01, /* 5 bNrInputPins : Number of Input Pins of this Jack. (field size 1 bytes) */
0x01, /* 6 BaSourceID(1) : ID of the Entity to which this Pin is connected. (field size 1 bytes) */
0x01, /* 7 BaSourcePin(1) : Output Pin number of the Entity to which this Input Pin is connected. */
USB_MIDI_TO_ESP_JACK_STR_INDEX, /* 5 iJack : Unused. (field size 1 bytes) */
/* Table B-11: MIDI Adapter Standard Bulk OUT Endpoint Descriptor */
0x09, /* 0 bLength : Size of this descriptor, in bytes. (field size 1 bytes) */
0x05, /* 1 bDescriptorType : ENDPOINT descriptor. (field size 1 bytes) */
USB_ENDPOINT_NUMBER_MIDI_TO_HOST, /* 2 bEndpointAddress : OUT Endpoint 3. (field size 1 bytes) */
0x02, /* 3 bmAttributes : Bulk, not shared. (field size 1 bytes) */
0x00, /* 4 wMaxPacketSize : 512 bytes per packet. (field size 2 bytes) - has to be 0x200 for compliance*/
0x02, /* 5 wMaxPacketSize */
0x00, /* 6 bInterval : Ignored for Bulk. Set to zero. (field size 1 bytes) */
0x00, /* 7 bRefresh : Unused. (field size 1 bytes) */
0x00, /* 8 bSynchAddress : Unused. (field size 1 bytes) */
/* Table B-12: MIDI Adapter Class-specific Bulk OUT Endpoint Descriptor */
0x05, /* 0 bLength : Size of this descriptor, in bytes. (field size 1 bytes) */
0x25, /* 1 bDescriptorType : CS_ENDPOINT descriptor (field size 1 bytes) */
0x01, /* 2 bDescriptorSubtype : MS_GENERAL subtype. (field size 1 bytes) */
0x01, /* 3 bNumEmbMIDIJack : Number of embedded MIDI IN Jacks. (field size 1 bytes) */
0x01, /* 4 BaAssocJackID(1) : ID of the Embedded MIDI IN Jack. (field size 1 bytes) */
/* Table B-13: MIDI Adapter Standard Bulk IN Endpoint Descriptor */
0x09, /* 0 bLength : Size of this descriptor, in bytes. (field size 1 bytes) */
0x05, /* 1 bDescriptorType : ENDPOINT descriptor. (field size 1 bytes) */
USB_ENDPOINT_NUMBER_MIDI_FROM_HOST, /* 2 bEndpointAddress : IN Endpoint 3. (field size 1 bytes) */
0x02, /* 3 bmAttributes : Bulk, not shared. (field size 1 bytes) */
0x00, /* 4 wMaxPacketSize : 512 bytes per packet. (field size 2 bytes) - has to be 0x200 for compliance*/
0x02, /* 5 wMaxPacketSize */
0x00, /* 6 bInterval : Ignored for Bulk. Set to zero. (field size 1 bytes) */
0x00, /* 7 bRefresh : Unused. (field size 1 bytes) */
0x00, /* 8 bSynchAddress : Unused. (field size 1 bytes) */
/* Table B-14: MIDI Adapter Class-specific Bulk IN Endpoint Descriptor */
0x05, /* 0 bLength : Size of this descriptor, in bytes. (field size 1 bytes) */
0x25, /* 1 bDescriptorType : CS_ENDPOINT descriptor (field size 1 bytes) */
0x01, /* 2 bDescriptorSubtype : MS_GENERAL subtype. (field size 1 bytes) */
0x01, /* 3 bNumEmbMIDIJack : Number of embedded MIDI OUT Jacks. (field size 1 bytes) */
0x03, /* 4 BaAssocJackID(1) : ID of the Embedded MIDI OUT Jack. (field size 1 bytes) */
},
};
Code: Select all
if(result == XUD_RES_ERR)
{
/* Return Audio 2.0 Descriptors with Null device as fallback */
result = USB_StandardRequests(ep0_out, ep0_in,
(unsigned char*)&devDesc_Audio, sizeof(devDesc_Audio),
(unsigned char*)&cfgDesc_Audio, sizeof(cfgDesc_Audio),
NULL, 0,
NULL, 0,
usb_stringDescriptors,
sizeof(usb_stringDescriptors) / sizeof(usb_stringDescriptors[0]), &sp,
usbBusSpeed);
}
My questions are:
Occurs the error during the enumeration process? If so, can you help me pinpoint where the descriptors are wrong?
Do you need any additional information?
Thanks for your help!!
Tim